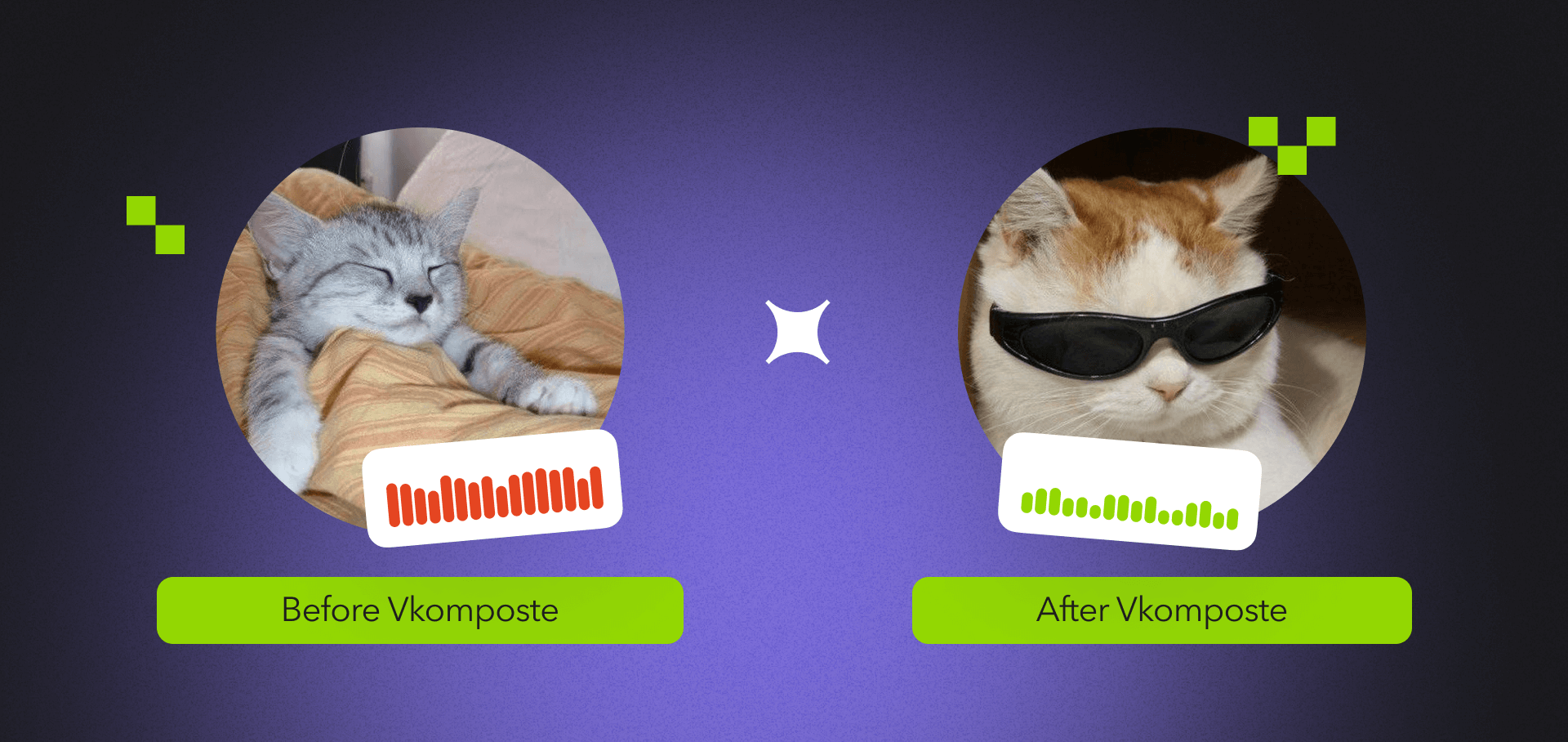
Jetpack Compose is a powerful UI framework that simplifies Android development. With its declarative approach, reduced code, and flexible state management, it enables faster and more efficient UI creation.
However, if used incorrectly, Jetpack Compose can have performance issues. Poor state management or resource overuse due to frequent redraws and complex animations can slow down an app.
Key Takeaways
- Jetpack Compose simplifies Android development but can face performance issues if not used properly, especially with poor state management and too many recompositions.
- Recomposition errors such as using unstable types, lambdas, and large recomposition areas can slow down the app.
- Vkompose provides plugins (IDEA, Gradle, and Detekt) that find and fix these issues by showing where things can be improved, logging recompositions, and preventing the build of unoptimized code.
- Recomposition logging helps track and fix performance bottlenecks in real-time, both on emulators and real devices.
- Vkompose works better than other tools like Android’s Layout Inspector and Rebugger by offering more flexible logging and better integration with CI/CD for smoother UI performance.
Common Jetpack Compose Optimization Mistakes
Recomposition is the process of redrawing UI components in Jetpack Compose, which can significantly impact performance. Every time the state of a component changes, partial or full recomposition can occur, leading to unnecessary UI redraws.
Key reasons for frequent recompositions include:
- Unstable Types
Using unstable data types, such as mutable collections or data classes, can trigger redundant recompositions in @Composable functions. Even minor state changes can cause the entire UI element to be redrawn. To avoid this, use stable types or annotate components with @Stable and @Immutable.
- Using Lambdas
Passing lambda functions as parameters to @Composable functions often results in unnecessary recompositions, as the compiler creates new objects with each call. It's better to use method references (e.g., ::functionName) to minimize redraws and improve performance.
- Large Recomposition Areas
When designing an interface, it's essential to break functions into smaller components to limit the recomposition scope. If one part of a function remains static while another changes frequently, it's better to split them into separate functions. This allows the frequently changing part to be redrawn independently, boosting efficiency.
Vkompose Features
Vkompose is a set of plugins that detect performance errors in @Composable functions during both compilation and execution.
- IntelliJ IDEA Plugin
Vkompose integrates as a plugin for IntelliJ IDEA and is available for all recent versions of Android Studio, excluding Canary builds. The plugin highlights @Composable features that are prone to missed recompositions and unstable parameters that may lead to extra redraws.

- Gradle Plugin
The full package of Vkompose Gradle plugins can be integrated, or they can be connected separately. This plugin performs checks at compile-time, ensuring that applications won't be built until errors are fixed or necessary annotations are added, preventing unoptimized code from being written.
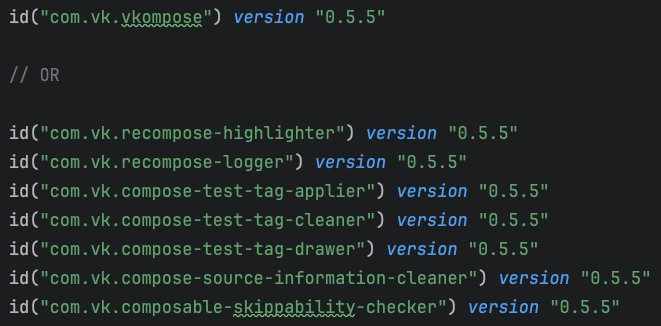
One key function is recomposition logging. It tracks recompositions of UI elements on the current screen, detailing the reason and data behind each new redraw:
MainActivity.kt:SampleScreen:Column:Text recomposed 1 times. Reason for now:
text changed: prev=[value=Some Name, hashcode = 1360724535], current=[value=num 1, hashcode = 105178647]
MainActivity.kt:SampleScreen:Column:Text recomposed 2 times. Reason for now:
text changed: prev=[value=num 1, hashcode = 105178647], current=[value=num 2, hashcode = 105178648]
MainActivity.kt:SampleScreen:Column:Text recomposed 3 times. Reason for now:
text changed: prev=[value=num 2, hashcode = 105178648]
You can manually add logs to track specific data using RecomposeLogger to log recompositions and identify causes for performance issues.
@Suppress("NonSkippableComposable")
@Composable
fun SampleScreen(
data: SomeData,
someEffects: Flow<SomeEffect>
) {
RecomposeLogger(
name = "SampleScreenLogger",
arguments = mapOf("data" to data)
)
Column {
Text(text = data.someName)
}
}
Result:
SampleScreenLogger recomposed 4 times. Reason for now:
data changed: prev=[value=SomeData(someName=num 3, sampleList=[]), hashcode = -1034429176], current=[value=SomeData(someName=num 4, sampleList=[]), hashcode = -1034429145]
SampleScreenLogger recomposed 5 times. Reason for now:
data changed: prev=[value=SomeData(someName=num 4, sampleList=[]), hashcode = -1034429145], current=[value=SomeData(someName=num 5, sampleList=[]), hashcode = -1034429114]
The plugin can highlight recompositions during code execution, whether on an emulator or a real device. This feature visually tracks components that slow down the UI, making it easier to identify and fix performance issues.
- Detekt Rules
Vkompose also integrates with Detekt, allowing developers to check for missing or unstable parameters during manual or CI/CD builds. This adds an extra layer of protection against unoptimized UIs reaching users.
Why Vkompose?
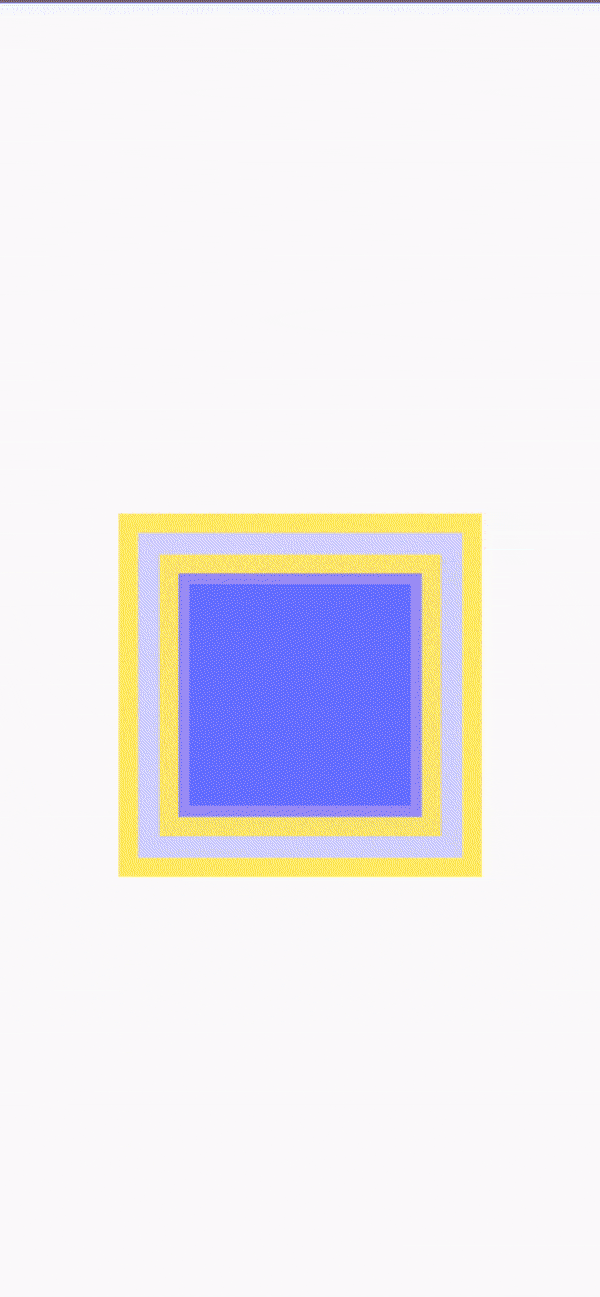
Vkompose isn’t the only tool for detecting unoptimized UI in Compose. Android's built-in Layout Inspector allows you to examine UI hierarchies and see recomposition counts, but this feature is limited to emulators. Vkompose, by contrast, combines highlighting and logging to efficiently find bugs and performance bottlenecks.
While Rebugger offers recomposition logging for specific components, Vkompose provides more flexibility, allowing logs for all components at once. In our experience, Rebugger sometimes skips recompositions, making it less reliable for detecting frequent updates.
Additionally, Vkompose excels in checking missing features and unstable parameters in CI/CD, something Rebugger and other tools like Detekt don’t handle as well.
Conclusion
Vkompose is a powerful tool for optimizing UI performance in Jetpack Compose, but it requires experienced developers who understand how to use it effectively. Combining Vkompose with skilled development ensures a stable, smooth UI in your Android app.
A stable and responsive UI is crucial for user retention in any mobile app. The smoother and more responsive the UI, the more likely users are to stay with the app rather than switch to another. Therefore, to ensure a positive user experience, it's important to focus not just on UI design, but also on its performance.
Interested in developing your own Android app or improve the existing one? Contact us or book a quick call for a free personal consultation and system audit.
Take a look at our other articles too:
What Is Code Auditing And How to Conduct It
Comments